最近在 Twitter 上看到很多人在发一种几个绿黄灰色 emoji 组成的推文,感觉像是 Github 的图但是有不太对,一查发现原来是一种叫 wordle 文字解密游戏,而推文就是每日题目的成绩。
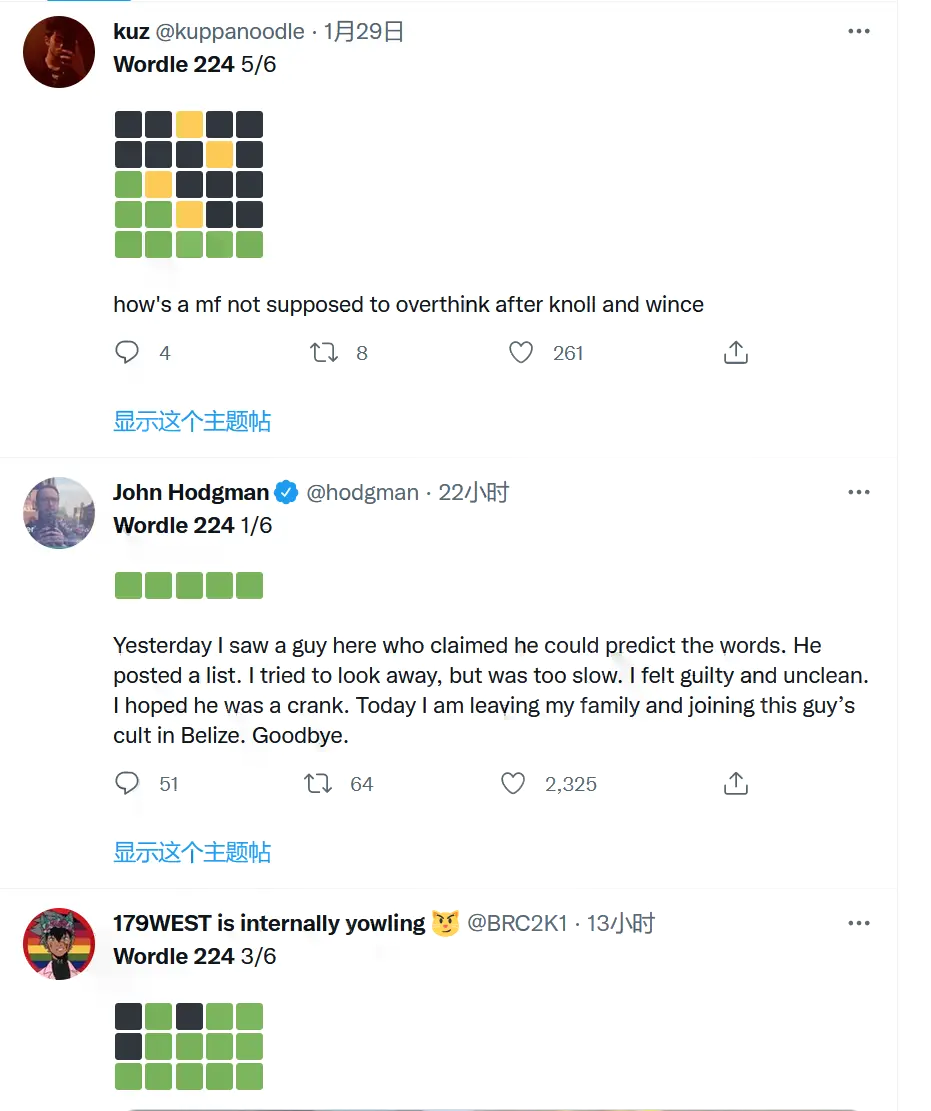
游戏的规则很简单,就是猜5个字母长度的单词,每天有6次机会,每次猜完都会告诉你猜的结果。
- 绿色: 这个方块的字母在正确的位置
- 黄色: 这个方块的字母在单词里但是位置是错误的
- 灰色: 单词中不包含这个方块的字母
思路很简单
- 找到所有的英文单词。
- 筛选出所有5个字母长度的单词。
- 去除所有包含灰色字母的单词
- 去除所有不包含黄色字母的单词。
- 去除所有绿色方块对应位置字母不一致的单词。
- 从剩余词库列表中验证
- 重复 3-6 过程。
效果还是不错的,基本可以在5步之内解出。
完整代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87
| from math import ceil from random import random
word_list = [] for line in open('engmix.txt','r',encoding='utf-8'): if len(line) == 6: word_list.append(line[0:5].lower())
print("在结果中输入5位数组") print("输入1代表绿色,输入2代表黄色,输入3代表灰色") print("Example:12331")
while True: print("----------------------------------------------------") print("候选单词数量:", len(word_list)) random_num = ceil(random() * len(word_list)) - 1 print("随机数:", random_num) if len(word_list) == 0: print("没有单词了!") exit() word = word_list[random_num] print("输入单词:", word) check = str(input('输入结果: ')) if check == 'r': continue white_list = set()
for index, select in enumerate(check): if select == '1': print("添加白名单:", word[index]) white_list.add(word[index])
for index, select in enumerate(check):
if select == '3': gary_word = word[index] counter = 0 while counter < len(word_list): if len(word_list) == counter: break if (gary_word in word_list[counter]) and (gary_word not in white_list): print("排除单词:",word_list[counter]) word_list.remove(word_list[counter]) else: counter += 1
elif select == '2': yellow_word = word[index] counter = 0 while counter < len(word_list): if len(word_list) == counter-1: print("break") break if (yellow_word not in word_list[counter]) or (yellow_word == word_list[counter][index]): print("排除单词:", word_list[counter]) word_list.remove(word_list[counter]) else: counter += 1
elif select == '1': green_world = word[index] counter = 0 while counter < len(word_list): if word_list[counter][index] != green_world: print("排除单词:", word_list[counter]) word_list.remove(word_list[counter]) counter -= 1 if len(word_list) == counter-1: break counter += 1
|